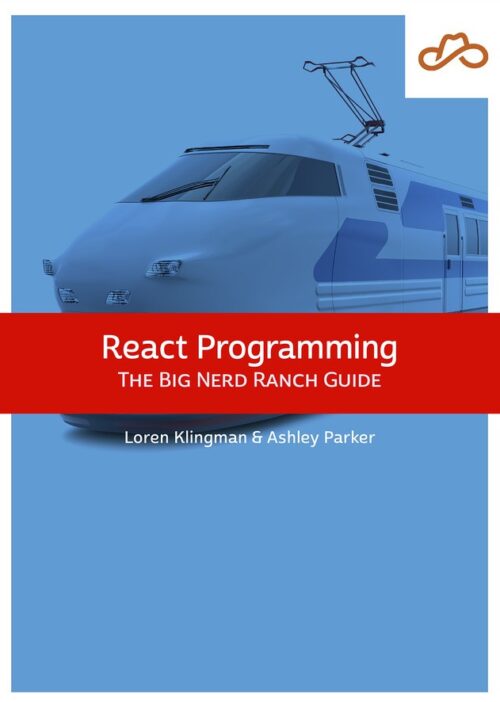
Now Available React Programming: The Big Nerd Ranch Guide
Front-End ReactBased on our React Essentials course, this book uses hands-on examples to guide you step by step through building a starter app and a complete,...
Editor’s Note: The API used by this blog series is no longer available. As a result, you will not be able to run this application locally, but you can read the code and explanations to see how to build a robust real-world frontend app data layer.
Even though most frontend apps are backed by a web service, building out the data later for such frontend apps is hard. State management libraries are often unopinionated about organizing your data, so you need to decide that for yourself. The state management libraries aren’t always designed with an eye toward accessing data from web services, so setting up that access can take some work. And although browsers now have good support for running apps offline, actually building a real system to do so is fraught with inherent complexity.
Some GraphQL clients such as Apollo have built-in support for both remote and local data, but you still need to make decisions about when and how remote and locally-cached data should interact. If you want to fully escape that complexity, there are a few off-the-shelf data libraries that handle much of this complexity for you—but their features, pricing, and data privacy won’t be a fit for every project.
With all these challenges, how can we efficiently set up robust data layers for our frontend apps? This blog post series is an attempt to answer this question by demonstrating common patterns for building a robust data layer in the context of a React app. The code we’ll build together can be used as the basis for a React/Redux app connecting to a JSON-based web service. The same patterns can be applied in other contexts as well, such as if you’re building an app with a GraphQL client, another frontend framework like Vue, or a native platform. And if you’re considering an off-the-shelf system like Firebase or Realm, these principles will help you evaluate the features they offer and think through any bugs that come up while integrating them.
We’ll apply these principles over the course of building out a project for tracking a list of video games. On the surface, the features couldn’t be simpler: we’ll display a list of video game titles and provide the ability to add additional games. We won’t even be building the ability to edit or delete games! But the apparent simplicity will highlight the depth of complexity under the surface, as we tackle questions like:
This series assumes you have familiarity with modern JavaScript features like:
If not, the above links go to excellent articles and chapters by Axel Rauschmayer introducing them. Familiarity with modern JavaScript features is an important way to be effective in React development in general and frontend development in particular, so it will be time well spent!
This series also assumes you have a basic familiarity with React, Redux, and connecting to web services (we’ll be using the Axios client library to do so). If not, spend some time with the following guides:
We’ll also be using the following libraries and formats, but it’s okay if you aren’t familiar with them. You’ll be able to pick up enough about how they work from how we use them in this guide, and you can dive into them more in-depth later as you have need.
Although I prefer and recommend Firefox for general web browsing, in this guide we’ll be using Google Chrome for some of the features its web developer tools provide when it comes to easily working with service workers for offline purposes.
You’ll also notice that we use the Yarn package manager in place of npm
. Yarn connects to the same NPM repository as the npm
client; it just provides simpler commands, better performance, and a more predictable use of lock files. We recommend using Yarn for all professional frontend projects.
React has a lot of different options for state management layers, and Redux isn’t the best choice for everything. Let’s talk through some of the options out there and why you might choose them.
To learn more about these and many other options, check out a blog post about React State Museum, a project to compare different React state management options.
So why are we going with Redux in this case? A few reasons:
That’s all the introduction we need. Check back on May 20 and we’ll get started creating our app!
Based on our React Essentials course, this book uses hands-on examples to guide you step by step through building a starter app and a complete,...
Svelte is a great front-end Javascript framework that offers a unique approach to the complexity of front-end systems. It claims to differentiate itself from...
Large organizations with multiple software development departments may find themselves supporting multiple web frameworks across the organization. This can make it challenging to keep...