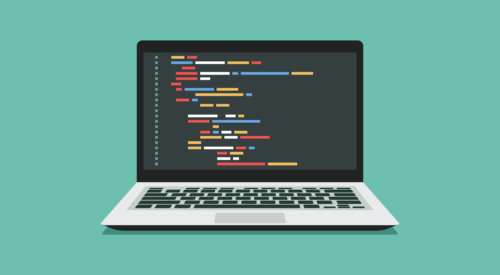
From Punched Cards to Prompts
AndroidIntroduction When computer programming was young, code was punched into cards. That is, holes were punched into a piece of cardboard in a format...
Today we are going to talk a bit about Gatsby.js and Netlify, what they are, and how they can be used.
Chances are you’ve probably heard about Gatsby.js. If you haven’t, here is a quick definition of Gatsby.js from their site:
Gatsby is a free and open source framework based on React that generates some truly righteous static sites with the added benefit of a plethora of plugins and starters.
The documentation is nicely written, well sourced, and communicates clearly: We are here to help. Of course — as with every framework, library, or otherwise categorized collection of code — there are always pitfalls, but the overall feeling of the framework itself suggests an ease of use and clearly defines well-handled abstractions.
As a nice, compact experiment to explore these new concepts, let’s build a tribute site to the roaring twenties with our new site generator.
With the assumption that you have already acquired some knowledge and have installed npm
and subsequently yarn
, we will begin. The gatsby documentation recommends a global installation of their CLI. The command line interface: gatsby-cli
, provides a bunch of useful commands that help make development smoother. Open your terminal and run this command:
$ yarn global add gatsby-cli
Or if you prefer npm
:
$ npm install gatsby-cli --global
Success! We now have the tool needed to create our tribute site. Let’s create it.
$ gatsby new tribute $ cd tribute $ gatsby develop
There it is, your new site, and it’s set up to establish good practices for loading static assets quickly and efficiently. It also has a GraphQL instance running in the background that lets you query your directory source tree, markdown pages and a host of other options. We’ll dig into our simple use case for querying the source tree later. For now, open the code in your favorite code editor, and check out the rendered front-end at localhost:8000
. You should see this:
Make a change, some text to start. You’ll notice that hot reloading refreshes your changes for you. Having a development environment is great, but the real question might be: where is production going?
For our production environment, we are going to set up a GitHub repo and link that GitHub repo to Netlify. Gatsby.js thoughtfully includes a .gitignore
in its default starter. Open up a new tab on GitHub, and create a new repository.
Gatsby also starts you out with a .git
folder to track changes, so no need to git init
. Just grab your new repo’s URL, and add the remote origin, package up your changes, and push to master.
$ git remote add origin <new_repo_url> $ git add -A $ git commit -m 'default gatsby boilerplate' $ git push -u origin master
Next step for production is to link your Gatsby.js site repository to Netlify. If you are looking for more dynamism, you may want to consider your options carefully before moving forward with Netlify. For now the ask is minimal and well defined: a simple static tribute site.
Step one: Make a Netlify account. Once that’s finished, make a new project. You should start out on the overview page. Underneath the header that announces your team and project name, there is some tab navigation:
Inside that navigation, click on Deploys -> Deploy Settings -> Link Site To Git
and link up your GitHub account to Netlify. You are looking for a button like this:
Choosing one of these options should allow you to search for a repository.
You might need to configure the Netlify app on GitHub if you haven’t already set up Netlify permissions. Netlify provides a link in the repository search screen. Find your repo, and select it to attach to the Netlify instance. Once attached, you should see a screen like this:
These are the defaults settings for Netlify. It means that your deployment hook, the action that listens for repo changes to rebuild your code, is attached to the master
branch. The script that is run when master
is updated is going to be gatsby build
, and the publish directory is going to be public
.
Unless you have some custom settings, and given the static site
nature of this build environment one would hope that isn’t the case, this setup should get you rolling.
Let’s get the deployment set, and then have some fun with the code. We already pushed to master, so no sense in doing it again. Instead, let’s manually trigger a rebuild from the Deploys
submenu:
Watch those logs for site is live
, then go to the remote path attached to your Netlify instance. You should see our default starter page up and running, purple astronaut and all.
At this point you might be chomping at the proverbial bit, wanting to get in there and really build something. Now is your time.
We are going to leverage some of fun, new libraries in our framework. The first one to explore out of the gate is the image service that Gatsby.js provides. It’s nicely compact, and will allow us to throw a hero image in there fairly quickly. Eventually, we will want to build more than one hero image onto our new page, so let’s gather a GraphQL
fragment together:
import { useStaticQuery, graphql } from "gatsby" ... export const heroQuery = graphql` fragment heroQuery on File { childImageSharp { fluid( maxWidth: 1240 ) { ...GatsbyImageSharpFluid } } } `
This is a standard GraphQL fragment, a reusable section of a query. It’s running against our GraphQL instance and is aware of the file tree. We’ll give it the name heroQuery
, and tell it that it’s going to search against the File
node.
The image library provides us with a fade-in effect to lazy-load
images. We could use the standard ...GatsbyImageSharpFluid
, however we have some more options. Appending the _tracedSVG
provides a very nice fade-in effect that traces the outline of the image as it lazy loads. It’s interesting for the viewer, and it helps with performance. Many thanks to Kyle Gill for the example ????.
export const heroQuery = graphql` fragment heroQuery on File { childImageSharp { sizes( maxWidth: 1240 ) { ...GatsbyImageSharpSizes_tracedSVG } } } `
It may be useful to note that GatsbyImageSharpSizes
won’t work without gatsby-transformer-sharp
and gatsby-plugin-sharp
. These libraries are generally packaged standard with our default loadout, but if you don’t see them you can run:
$ yarn add gatsby-transformer-sharp gatsby-plugin-sharp
Then, add them to your gatsby-config.js
. This allows you to directly access and set the options and transformers for the sharp image nodes library in Gatsby.js
module.exports = { plugins: [ `gatsby-transformer-sharp`, `gatsby-plugin-sharp`, ] }
Let’s take this fragment and add it to our boilerplate image
component. If you are wondering where that file is, check: ./src/components/
. This setup is pretty basic, we are using this GraphQL query and our hero fragment to look through our files and find our image.
import { useStaticQuery, graphql } from "gatsby" import Img from "gatsby-image" export const HeroImage = () => { const data = useStaticQuery(graphql` query { main: file(relativePath: { eq: "some-pic1.jpg" }) { ...heroQuery }, } `) return <Img title="The Roaring Twenties in Image Form" alt="Some awesome twenties stuff happening" sizes={data.main.childImageSharp.sizes} /> }
These queries operate through the GraphQL instance and are aware of the file path through the plugin attached in the gatsby-config.js
.
{ resolve: `gatsby-source-filesystem`, options: { name: `images`, path: `${__dirname}/src/images`, }, },
All pretty cool stuff. At this point I’m gonna do some hand waving (feel free to do your own dev magic) ????, get a few fonts and throw a bit of css
in there ????, to round things off ????. With all our new code in place, we package it up, push to master, and Netlify swings into action.
Deployed in a single push and production is golden, just like the age. If you liked this or found it helpful be sure to give us a shout. If you’re interested in the code base, check it out here.
Introduction When computer programming was young, code was punched into cards. That is, holes were punched into a piece of cardboard in a format...
Connecting the dots: how we got here At Big Nerd Ranch, we have some strongly held beliefs about how people best learn new technical...
Mac and Mobile – how it all started Big Nerd Ranch has made our name in Mac and mobile. We were the first to...