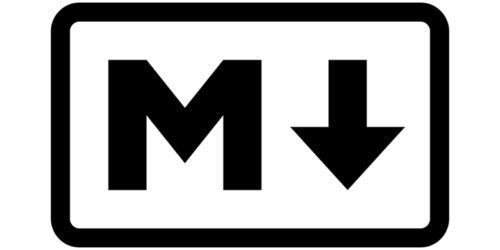
Four Key Reasons to Learn Markdown
Back-End Leveling UpWriting documentation is fun—really, really fun. I know some engineers may disagree with me, but as a technical writer, creating quality documentation that will...
In this series, we’ve managed to build a mobile app that will show us live notifications of events on services like GitHub using WebSockets. It works like this:
This is pretty great when we’re running the app. But what about when it’s in the background? In that case, we can use device push notifications to alert the user to events. Here’s the complete architectural diagram of the system, with push notifications added:
Push notifications are available on the web as well (but, as of the time of this writing, not on Safari). However, users are frequently less willing to enable push notifications in web apps than in native mobile apps. Because of this, we chose to build our client app in React Native using Expo. Expo has great support for push notifications across both iOS and Android—let’s give them a try!
If you like, you can download the completed server project and the completed client project for the series.
Before we can send a push notification to a user, we need to request permission from them. In our Expo app, in src/MainScreen.js
, add a new PushPermissionRequester
component:
import React from 'react'; import { View } from 'react-native'; +import PushPermissionRequester from './PushPermissionRequester'; import MessageList from './MessageList'; export default function MainScreen() { return ( <View style={{ flex: 1 }}> + <PushPermissionRequester /> <MessageList /> </View> ); }
Now let’s implement PushPermissionRequester
. Create a src/PushPermissionRequester.js
file and enter the following:
import React, { useState } from 'react'; import { View } from 'react-native'; import { Notifications } from 'expo'; import * as Permissions from 'expo-permissions'; import { Button, Input } from 'react-native-elements'; const askForPushPermission = setToken => async () => { }; export default function PushPermissionRequester() { const [token, setToken] = useState('(token not requested yet)'); return ( <View> <Input value={token} /> <Button title="Ask Me for Push Permissions" onPress={askForPushPermission(setToken)} /> </View> ); }
This component tracks a push notification token that can be requested, then is displayed afterward. Now let’s fill in askForPushPermission
to request it:
const askForPushPermission = setToken => async () => { const { status: existingStatus } = await Permissions.getAsync( Permissions.NOTIFICATIONS, ); let finalStatus = existingStatus; if (existingStatus !== 'granted') { const { status } = await Permissions.askAsync(Permissions.NOTIFICATIONS); finalStatus = status; } console.log('push notification status ', finalStatus); if (finalStatus !== 'granted') { setToken(`(token ${finalStatus})`); } let token = await Notifications.getExpoPushTokenAsync(); setToken(token); };
This is boilerplate code from the Expo Push Notification docs; what’s happening is:
Reload the Expo app on your virtual device, and tap on “Ask Me for Push Permissions.” You should see the message “(token undetermined),” and a yellow box error at the bottom of the screen. The error says “Error: Must be on a physical device to get an Expo Push Token.”
Time to take this app to your real phone!
On Android, there are a few different ways to get the app running on your physical device. On iOS things are a bit more locked down. Let’s look at the approach that will work for both iOS and Android.
On your phone, search for “Expo” in the App Store or Google Play Store, respectively. This is a client app from Expo that allows you to run your app before going through the whole app publishing process, which is a nice speed boost. Download the Expo app. If you haven’t already created a free Expo account, create one. Then log in to the Expo app.
Now we need to get our React Native application published to Expo so we can load its JavaScript assets into the Expo app. Open the Metro Bundler browser tab that Expo opens when you run it. In the sidebar, click “Publish or republish project…”:
Choose a unique “URL Slug” for your app, then click “Publish project.”
Expo will take a minute or two to bundle up your JavaScript and upload it. Ultimately you should get a box at the bottom-right of the browser window saying “Successfully published to…”
Reopen the Expo app on your phone, go to the Profile tab, and in the “Published Projects” list you should see your app. Tap on it, and it should open and display the initial data from Heroku.
Now, tap “Ask Me for Push Permissions” again, and give permission. This time, on a physical device, it should work!
You should see a token that looks like ExponentPushToken[…]
, with a string of letters and numbers in between the square brackets. This is a token that uniquely identifies your app running in Expo on your device. You can use this to hit Expo’s API to send a push notification.
Select the whole token, copy it, and transfer it to your development computer somehow. Emailing yourself is always an option if nothing else!
Before we code anything, we can test this push notification out through Expo’s Push Notifications Tool. Make sure Expo is in the background on your phone. Then, on your development machine, go to the Push Notifications Tool.
Paste your full token including the string ExponentPushToken
into the “Expo Push Token” field. For “Message Title,” type something.
Scroll to the bottom of the page and click “Send a notification”. A push notification should appear on your phone from the Expo app, displaying the title you entered.
Feel free to play around with other fields in the push notification tool as well.
Now that we have a token, we can provide it to our backend. In a production application, you would set up a way for each user to send that token up to the server and store it with their user account. Since user accounts aren’t the focus of our tutorial, we’re just going to set that token via an environment variable instead.
In our node app, in .env.sample
, add the following line:
CLOUDAMQP_URL=fake_cloudamqp_url +EXPO_PUSH_TOKEN=fake_expo_push_token MONGODB_URI=fake_mongodb_uri
In .env
add your token, filling in the real value. This is the value we wanted to keep out of our git repo; you don’t want me to find your push token and send spam to you!
CLOUDAMQP_URL=amqp://localhost +EXPO_PUSH_TOKEN=ExponentPushToken[...] MONGODB_URI=mongodb://localhost:27017/nodeifier
Next, add Expo’s SDK as a dependency to your Node app:
$ yarn add expo-server-sdk
As we did with MongoDB and RabbitMQ, let’s wrap Expo’s SDK in a module of our own, to hide it from the rest of our app. Create a lib/expo.js
file and add the following:
const Expo = require('expo-server-sdk').default; const token = process.env.EXPO_PUSH_TOKEN; const expo = new Expo(); async function push({ text }) { if (!Expo.isExpoPushToken(token)) { console.error(`Push token ${token} is not a valid Expo push token`); return; } const messages = [ { to: token, title: text, }, ]; console.log('sending to expo push', messages); const chunks = expo.chunkPushNotifications(messages); for (let chunk of chunks) { try { let ticketChunk = await expo.sendPushNotificationsAsync(chunk); console.log(ticketChunk); } catch (error) { console.error(error); } } } module.exports = { push };
We export a push
function that our app can use to send a push notification. We only use the text
field of the message. First, we get the Expo push token from the environment variable and confirm it’s valid. Then we construct a message object with the structure Expo’s Push Notification SDK expects. The SDK is set up to allow sending push notifications in batches, which is a bit overkill in our case, but we work with it. We log the success or error message just in case.
Now let’s send out a push notification from our worker. In an earlier part we mentioned that you could conceivably separate different webhook endpoints into different microservices or lambda functions for scalability. You could do the same thing with workers. But since we’re hosting on Heroku, which will give us one web dyno and one worker dyno for free, we’ll keep our worker code in a single worker process that is watching multiple queues.
How should we organize this one worker service with multiple concerns? Currently our worker is very small, so adding code to monitor a second queue to the same file wouldn’t clutter it up much. But for the sake of illustrating how to separate concerns, let’s refactor our worker into separate modules.
Create a workers/incoming.js
file, and copy and paste the require
and handleIncoming
code from workers/index.js
into it. Then export the handler:
const queue = require('../lib/queue'); const repo = require('../lib/repo'); const handleIncoming = message => repo .create(message) .then(record => { console.log('Saved ' + JSON.stringify(record)); return queue.send('socket', record); }); module.exports = handleIncoming;
Update workers/index.js
to import that function instead of duplicating it:
if (process.env.NODE_ENV !== 'production') { require('dotenv').config(); } const queue = require('../lib/queue'); -const repo = require('../lib/repo'); - -const handleIncoming = message => - repo - .create(message) - .then(record => { - console.log('Saved ' + JSON.stringify(record)); - return queue.send('socket', record); - }); +const handleIncoming = require('./incoming'); queue .receive('incoming', handleIncoming)
Now, where should we call our push
function? In this case, we could probably do it directly in handleIncoming
. But when you’re using a queue-based architecture it can be valuable to separate units of work into small pieces; that way if one part fails it can be retried without retrying the entire process. For example, if we can’t reach Expo’s push notification service, we don’t want a retry to inadvertently insert a duplicate record into our database.
So instead, let’s create a new push
queue that will receive messages each time we have a push notification to send. In workers/incoming.js
, just like we send a message to the socket
queue, we’ll send one to the push
queue as well:
const handleIncoming = message => repo .create(message) .then(record => { console.log('Saved ' + JSON.stringify(record)); - return queue.send('socket', record); + return Promise.all([ + queue.send('socket', record), + queue.send('push', record), + ]); });
Note that we wrap our two queue.send
calls in a Promise.all()
and return the result; that way if either of the sends fails, the rejection will be propagated up and eventually logged with console.error
.
Next, add a new workers/push.js
file with the following contents:
const expo = require('../lib/expo'); const handlePush = message => { console.log('handling push', message); return expo.push(message); }; module.exports = handlePush;
An extremely simple worker, this just forwards the received message along to our Expo module. Connect it in workers/index.js
:
const queue = require('../lib/queue'); const handleIncoming = require('./incoming'); +const handlePush = require('./push'); queue .receive('incoming', handleIncoming) .catch(console.error); +queue + .receive('push', handlePush) + .catch(console.error);
With this, we should be set up to send push notifications. Run your two node processes locally:
$ node web
$ node workers
Send a test notification:
$ curl http://localhost:3000/webhooks/test -d "this should be pushed"
You should see the push notification show up on your phone. Note that although your Expo app is pointing to your production server, it still receives the push notification from your local server. This is because we’re using your device’s Expo push token, and it doesn’t know or care about any other backing servers.
Our final step is to get push notifications working in production. Whereas our previous Heroku environment variables were provided for us by add-ons, we need to set our EXPO_PUSH_TOKEN
variable manually. There are two ways we can do this:
heroku config:set "EXPO_PUSH_TOKEN=ExponentPushToken[...]"
(entering your full token as usual)EXPO_PUSH_TOKEN
for the KEY and your token for the VALUE, then click Add.Commit your latest changes then push them to Heroku:
$ git add . $ git commit -m "updated for push tokens" $ git push heroku master
When your app finishes deploying, try sending it a webhook, filling in your app’s URL instead of mine:
$ curl https://murmuring-garden-42327.herokuapp.com/webhooks/test -d "push from production"
You should receive a push notification on your phone.
You can also try toggling your GitHub PR to see that your other webhooks also deliver push notifications now too.
With that, our app is complete! Let’s review what we’ve built one last time:
We’ve been able to hook up to live updates coming from services like GitHub, Heroku, and Netlify. We set up a queue-based architecture to ensure that on real systems that would have far more load than this, that each piece of the process can run performantly. We push data to running apps over WebSockets, and apps in the background using push notifications.
Adding live updates to your mobile or web applications using approaches such as these can be a big boost to your app’s usefulness to your users. If you’re a developer, give these technologies a try. And if Big Nerd Ranch could help train you in these technologies or help build the foundation of a new live-updating application for you, let us know!
Writing documentation is fun—really, really fun. I know some engineers may disagree with me, but as a technical writer, creating quality documentation that will...
Humanity has come a long way in its technological journey. We have reached the cusp of an age in which the concepts we have...
Go 1.18 has finally landed, and with it comes its own flavor of generics. In a previous post, we went over the accepted proposal and dove...