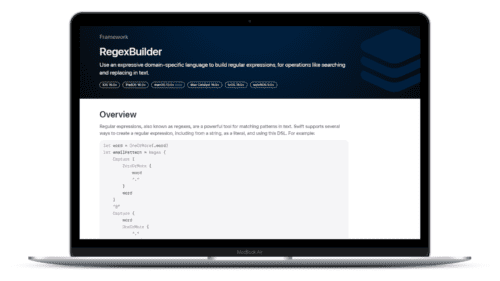
Swift Regex Deep Dive
iOS MacOur introductory guide to Swift Regex. Learn regular expressions in Swift including RegexBuilder examples and strongly-typed captures.
The UI in iOS 11 is mostly unchanged from that of iOS 10, but it does add a new variation to the navigation bar.
iOS 11 added a property to UINavigationItem
that enables a large, out-of-line title in the navigation bar. Apple recommends that this larger title be enabled for the first view controller in a navigation controller, but you can use it with any view controller.
To display a large title, set the navigation item’s largeTitleDisplayMode
to .always
:
let vc = UIViewController()
vc.navigationItem.largeTitleDisplayMode = .always
Yes, that’s pretty much it. Now our app went from this:
to this:
Oh no, something went wrong. This is what happens when the offset for the content was hardcoded; the taller title breaks the assumed fixed height, and we get unwanted overlap. If we’d used topLayoutGuide
, then we’d be fine. But iOS 11 also deprecates topLayoutGuide
!
safeAreaLayoutGuide
replaces topLayoutGuide
and bottomLayoutGuide
as a new way to create constraints between your views and a superview. These guides are used in tvOS to help insure that important areas of your view aren’t cut off because of overscan. These guides can also be used as new anchors for your views within a UIViewController
to make sure that the UINavigationBar
or UITabBar
isn’t blocking your content.
If you are familiar with writing programmatic constraints, using these new guides couldn’t be easier. Let’s see if we can fix our app using these new layout guides:
topLabel.topAnchor.constraint(
- equalTo: view.topAnchor,
- constant: 64
+ equalTo: view.safeAreaLayoutGuide.topAnchor
).isActive = true
With that fix, our content moves back beneath even the large-titled Navigation Bar:
If your constraints were created through Interface Builder, you can also opt into using the new layout guides. Open your Storyboard, view the File Inspector and select “Use Safe Area Layout Guides.”
Whether your project uses hardcoded offsets or topLayoutGuide
and bottomLayoutGuide
, we recommend using safeAreaLayoutGuide
.
Learn more about why you should update your apps for iOS 11 before launch day, or download our ebook for a deeper look into how the changes affect your business.
Our introductory guide to Swift Regex. Learn regular expressions in Swift including RegexBuilder examples and strongly-typed captures.
The Combine framework in Swift is a powerful declarative API for the asynchronous processing of values over time. It takes full advantage of Swift...
SwiftUI has changed a great many things about how developers create applications for iOS, and not just in the way we lay out our...