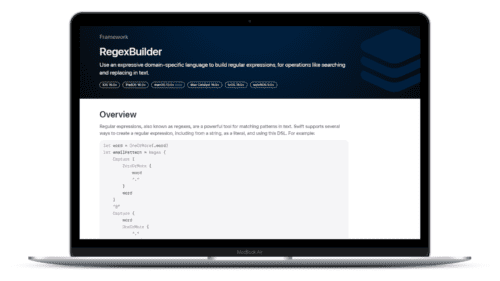
Swift Regex Deep Dive
iOS MacOur introductory guide to Swift Regex. Learn regular expressions in Swift including RegexBuilder examples and strongly-typed captures.
Drag and Drop makes it easier to get work done on an iPad by allowing you to drag links, text, images and documents between apps in a way that was never possible. For example, creating a note in the Notes app with text and photos from a website was a tedious task composed of copying and pasting each item. With Drag and Drop, I am able to grab multiple types of items at once from the webpage and drop them all at once in my note.
Drag and Drop drags content from a source and drops it on a target. A view becomes a drag source by implementing the UIDragInteractionDelegate
protocol. A view becomes a drop target by implementing the UIDropInteractionDelegate
protocol.
And the good news is, it’s easy to implement.
UIDragInteractionDelegate
.UIDragInteraction
instance and add it to your view.dragInteraction(_:itemsForBeginning:)
method in the delegate.
let dragInteraction = UIDragInteraction(delegate: self)
view.addInteraction(dragInteraction)
func dragInteraction(_ interaction: UIDragInteraction, itemsForBeginning session: UIDragSession) -> [UIDragItem] {
guard let image = imageView.image else { return [] }
let provider = NSItemProvider(object: image)
let item = UIDragItem(itemProvider: provider)
item.localObject = image
return [item]
}
That’s it! You can now drag your item around your view and across views. Next, you need to implement Drop.
UIDropInteractionDelegate
on the view you want to accept drops.UIDropInteraction
.dropInteraction(_ interaction:, canHandle session:) -> Bool
method.dropInteraction(_ interaction:, sessionDidUpdate session:) -> UIDropProposal
method (copy, move, etc.).dropInteraction(_ interaction:, performDrop session:)
. let dropInteraction = UIDropInteraction(delegate:self)
view.addInteraction(dropInteraction)
func dropInteraction(_ interaction: UIDropInteraction, canHandle session: UIDropSession) -> Bool {
return session.canLoadObjects(ofClass: [UIImage.self])
}
func dropInteraction(_ interaction: UIDropInteraction, sessionDidUpdate session: UIDropSession) -> UIDropProposal {
return UIDropProposal(operation: .copy)
}
func dropInteraction(_ interaction: UIDropInteraction, performDrop session: UIDropSession) {
session.loadObjects(ofClass: UIImage.self) { imageItems in
let images = imageItems as! [UIImage]
self.imageView.image = images.first
}
}
To allow your apps to send or receive data from other apps, implement the designated delegate protocol and follow the steps above for that protocol. There are many more methods that can be implemented to give greater customization to the drag and drop workflow in Apple’s documentation.
With all of the talk about iPad, you may be wondering if this feature is available on the iPhone. The answer is yes and no. While the full API is available on the iPhone, it only works within individual apps. You cannot drag items from one app to another, but you can, for example, drag a file from one folder in your app to another folder in another part your app.
In 2015, Apple took iPad to a whole other level with multitasking. Now, with Drag and Drop, Apple continues to push iPad further. You now know how easy it is to implement this powerful feature into your apps. As an iPad user, I can’t wait to see what you and the rest of developers come up with using Drag and Drop.
Learn more about why you should update your apps for iOS 11 before launch day, or download our ebook for a deeper look into how the changes affect your business.
Our introductory guide to Swift Regex. Learn regular expressions in Swift including RegexBuilder examples and strongly-typed captures.
The Combine framework in Swift is a powerful declarative API for the asynchronous processing of values over time. It takes full advantage of Swift...
SwiftUI has changed a great many things about how developers create applications for iOS, and not just in the way we lay out our...