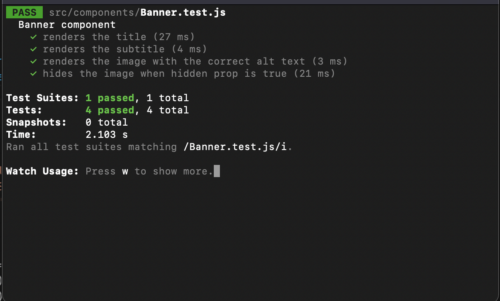
Replacing Myself: Writing Unit Tests with ChatGPT
Leveling UpThe Bot that’s got everyone talking The science-fiction future is among us as we find ourselves on the precipice of an AI revolution. As...
Do you use Google Chrome on a Mac? Do you ever find yourself with multiple browser tabs open? Or multiple browser windows each with multiple tabs open? Do you ever wish you could close a particular browser window or group of browser windows and restore them later?
Features like Google Chrome’s tab groups allow you to organize your tabs within a window. Tab groups can also be expanded or collapsed as you work on different groups of tabs throughout the day.
Continue reading if you’d like to learn how to further organize your workflow with separate browser sessions that you can close and restore using a shell script from the command line.
At Big Nerd Ranch I often find myself working on multiple tasks throughout the week. I found myself with multiple browser windows open, each with multiple tabs open. Each browser window represented some unit of work I was working on. Sometimes a group of windows represented a unit of work. As the number of browser windows grew, it became hard to find the window or group of windows I needed when I needed them.
This challenge led me to explore options on how to start Google Chrome from the command line on my Mac. My goal was to be able to start, save, and restore named browser sessions that were easy to find.
The code for this script is available on GitHub at BNR-Developer-Sandbox/BNR-blog-chrome-management.
Here’s where I landed on the script:
#!/bin/bash WORKING_ON=$1; # get directory name from first command line argument # Create working directory and error log if needed mkdir -p ${WORKING_ON}; touch ${WORKING_ON}/error.log; echo "Working on... ${WORKING_ON}"; echo "Google Chrome error log at ./${WORKING_ON}/error.log"; echo "Use ^C to close your browser session."; # Create or Restore a Google Chrome session based on what you are working on # Redirect Google Chrome output errors to error.log /Applications/Google\ Chrome.app/Contents/MacOS/Google\ Chrome \ --user-data-dir="./${WORKING_ON}" --restore-last-session \ 2>> ./${WORKING_ON}/error.log; GOOGLE_CHROME_EXIT=$?; # capture Google Chrome exit code echo; if [ $GOOGLE_CHROME_EXIT -eq 0 ] then echo "Google Chrome Session saved: ${WORKING_ON}"; else echo "Google Chrome exited with error code: ${GOOGLE_CHROME_EXIT}."; echo "See ./${WORKING_ON}/error.log for error details."; fi echo "Run rm -r ./${WORKING_ON} to permanently delete this session." echo "Re-run ./work-on.sh ${WORKING_ON} to restore this session."; exit $GOOGLE_CHROME_EXIT;
To install the script, you can clone the repository:
$ git clone git@github.com:BNR-Developer-Sandbox/BNR-blog-chrome-management.git Browsers Cloning into 'Browsers'... remote: Enumerating objects: 26, done. remote: Counting objects: 100% (26/26), done. remote: Compressing objects: 100% (25/25), done. Receiving objects: 100% (26/26), 4.24 KiB | 2.12 MiB/s, done. remote: Total 26 (delta 8), reused 2 (delta 0), pack-reused 0 Resolving deltas: 100% (8/8), done.
Then, change into the Browsers
directory where the work-on.sh
script is found:
$ cd Browsers
Now you can execute work-on.sh
to run the script and use ^C
to close the script:
$ ./work-on.sh BNR Working on... BNR Google Chrome error log at ./BNR/error.log Use ^C to close your browser session. ^C Google Chrome Session saved: BNR Run rm -r ./BNR to permanently delete this session. Re-run ./work-on.sh BNR to restore this session.
I had way too many browser windows open. Each with multiple tabs. Each window represented a different project I was working on. Sometimes I’d have multiple windows open for a project.
My current list of projects includes long-running projects like:
That’s 7 browser windows so far that I need throughout the week or come back to periodically as needed.
In addition to those long-running projects, I also have more finite initiatives like:
That’s 17 more browser windows to sort through! 😱
I was minimizing browser windows for the projects I wasn’t working on at the moment and my dock began to look cluttered with browser windows.
I have a similar problem on my home computer as I have browser windows open for communication, art, music, and various other projects.
I knew I could start Google Chrome from the command line and that there were command-line options I could pass to it. I began my research by reading:
I searched through the list of command-line options and experimented until I found that --user-data-dir
and --restore-last-session
met my needs. --user-data-dir
allows you to specify a directory name to save session data. --restore-last-session
restores the session saved in the directory specified by --user-data-dir
.
I started from the command line and then encapsulated the workflow in a simple shell script. This example is implemented on a Mac. See the resources linked above if you’d like to alter this for another operating system. If you are using a different browser, you’ll need to change the path to your browser and update the command line options to match what your browser expects in the work-on.sh
shell script.
The only variable needed was the project name. I didn’t want to remember the command line options I needed or type out the long command each time. So, I wrote the script above to encapsulate what I learned and provide some useful output.
The script starts by saving the first command-line argument, the name of the project you are working on, in a variable called WORKING_ON
.
WORKING_ON=$1; # get directory name from first command line argument
The WORKING_ON
variable is used to create the directory for the browser session data and an error.log
.
# Create working directory and error log if needed mkdir -p ${WORKING_ON}; touch ${WORKING_ON}/error.log;
Next, the script starts Google Chrome with the desired command-line options for saving and restoring your session.
# Create or Restore a Google Chrome session based on what you are working on # Redirect Google Chrome output errors to error.log /Applications/Google\ Chrome.app/Contents/MacOS/Google\ Chrome \ --user-data-dir="./${WORKING_ON}" --restore-last-session \ 2>> ./${WORKING_ON}/error.log;
The script also redirects error output from Google Chrome to error.log
in your ${WORKING_ON}
directory.
This was done to remove visual noise from recurring error messages that were being printed out to the terminal. If you examine error.log
you might find messages about checking Bluetooth availability and default browser status.
When Google Chrome exits successfully, the script will print out that your session was saved. If Google Chrome exits with an error, the error code and log path will be printed out to the terminal.
GOOGLE_CHROME_EXIT=$?; # capture Google Chrome exit code echo; if [ $GOOGLE_CHROME_EXIT -eq 0 ] then echo "Google Chrome Session saved: ${WORKING_ON}"; else echo "Google Chrome exited with error code: ${GOOGLE_CHROME_EXIT}."; echo "See ./${WORKING_ON}/error.log for error details."; fi
Next, the script will print out some additional tips to remove your session data or restore your session.
echo "Run rm -r ./${WORKING_ON} to permanently delete this session." echo "Re-run ./work-on.sh ${WORKING_ON} to restore this session.";
Finally, the script will exit with the exit code returned by Google Chrome.
exit $GOOGLE_CHROME_EXIT;
In your terminal, clone the BNR-Developer-Sandbox/BNR-blog-chrome-management git repository and specify the directory name where you’d like to save your browser sessions. In this example, you’ll clone the repository to a directory called Browsers
.
git clone git@github.com:BNR-Developer-Sandbox/BNR-blog-chrome-management.git Browsers
Next, change into the Browsers
directory.
cd Browsers/
From here you will execute the work-on-sh
script.
Run ./work-on.sh BNR
to create a new browser session in a directory called BNR
.
The command will print out some information about what you are working on and how to close your browser session from the terminal.
$ ./work-on.sh BNR Working on... BNR Google Chrome error log at ./BNR/error.log Use ^C to close your browser session.
Google Chrome will start a new instance and you’ll notice the icon bouncing in your dock.
Click on the new Google Chrome icon and you’ll see a welcome prompt. Choose your default browser and usage statistic settings and click the “Start Google Chrome” button.
You’ll now have a new browser window open at chrome://welcome
.
Since we are working on BNR
at the moment, open a tab to our homepage and forums.
When you are done with your browser session you can quit Google Chrome from the menu Chrome > Quit Google Chrome
or using the ⌘Q
keyboard shortcut. You can also quit the broswer session with ^C
from your terminal which is the approach I use.
^C Google Chrome Session saved: BNR Run rm -r ./BNR to permanently delete this session. Re-run ./work-on.sh BNR to restore this session.
When you are ready to work on your BNR
project again, use ./work-on.sh BNR
to restore your browser session.
Google Chrome will pick up where you left off. If you used multiple browser windows in your session, they will all be restore. Your active tabs and scroll positions will also be restored.
Since the only argument to the work-on.sh
script is a directory name, you get tab completion out of the box. I suggest using meaningful prefixes to group directories together, this will complement tab completion well. For example, I have three different browser sessions for blog posts I’m working on. Each of these sessions is prefixed with Blog-
followed by a hyphen separated project name.
When you are done with a project and you no longer need to restore it, you can remove the session by deleting the related directory name. In this example you can run rm -r ./BNR
from your terminal to recursively remove the session directory.
Maybe you’d like to use a different browser or you aren’t working on a Mac. If that’s the case, then you’ll need to modifty the script to meet your needs. Research how to start your desired browser from the terminal and what command line options you’ll need. Modify the work-on.sh
script to meet your needs accordingly. Happy scripting! 😊
Using this small shell script helps me stay focused and pick up where I left off. If you are new to shell scripting, I hope this example is a good introduction. I hope you found this workflow useful.
The Bot that’s got everyone talking The science-fiction future is among us as we find ourselves on the precipice of an AI revolution. As...
Big Nerd Ranch is chock-full of incredibly talented people. Today, we’re starting a series, Tell Our BNR Story, where folks within our industry share...
Writing documentation is fun—really, really fun. I know some engineers may disagree with me, but as a technical writer, creating quality documentation that will...