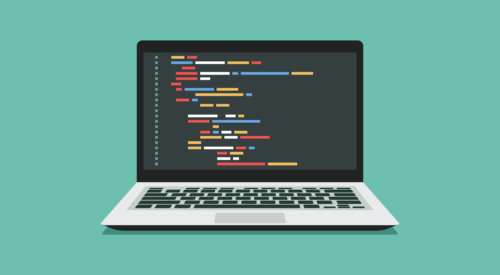
From Punched Cards to Prompts
AndroidIntroduction When computer programming was young, code was punched into cards. That is, holes were punched into a piece of cardboard in a format...
Every once in a while, an Android developer might want to use a ViewPager without the added complexity of also using Fragments. A good example is an image gallery, where the user can swipe between different pictures. On these types of pages, all you really want to display is a view of static content (in this case, an image), so I’m going to walk you through how to utilize the ViewPager with just plain-old Views and layouts.
Your journey begins with laying some foundational work with an XML layout, a build.gradle
file and the Activity that the ViewPager will live in.
Here’s your XML layout for the Activity, which consists solely of a ViewPager.
<android.support.v4.view.ViewPager
android:id="@+id/viewpager"
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
Don’t forget to add the support library as a gradle dependency. In newer versions of Android Studio, this should be added automatically at project creation.
dependencies {
compile 'com.android.support:support-v4:22.0.0'
}
Next you setup an Activity:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ViewPager viewPager = (ViewPager) findViewById(R.id.viewpager);
viewPager.setAdapter(new CustomPagerAdapter(this));
}
}
Nothing too exciting here, just grabbing a reference to your ViewPager and then setting its adapter to the super-special CustomPagerAdapter that will be covered later. This initial setup could also be done in a Fragment’s onCreateView()
method.
Need to know how to keep your development project on track and in budget? Download your eBook.
Personally, I like to have a model object representing the possible pages in the ViewPager. The below enum is essentially a listing of all of your ViewPager’s pages, with tidbits of information about each one thrown in, such as the page title and the layout to be shown. In reality, this could contain any information specific to a particular screen in your ViewPager.
public enum CustomPagerEnum {
RED(R.string.red, R.layout.view_red),
BLUE(R.string.blue, R.layout.view_blue),
ORANGE(R.string.orange, R.layout.view_orange);
private int mTitleResId;
private int mLayoutResId;
CustomPagerEnum(int titleResId, int layoutResId) {
mTitleResId = titleResId;
mLayoutResId = layoutResId;
}
public int getTitleResId() {
return mTitleResId;
}
public int getLayoutResId() {
return mLayoutResId;
}
}
Again, this enum isn’t strictly necessary, but it helps organize the separation of the content (the actual screen being shown) and the controller (the ViewPager).
The PagerAdapter is the core piece of this exercise. Everything that you’ve done so far is to make your life a bit easier when dealing with the PagerAdapter. So, here it is:
public class CustomPagerAdapter extends PagerAdapter {
private Context mContext;
public CustomPagerAdapter(Context context) {
mContext = context;
}
@Override
public Object instantiateItem(ViewGroup collection, int position) {
CustomPagerEnum customPagerEnum = CustomPagerEnum.values()[position];
LayoutInflater inflater = LayoutInflater.from(mContext);
ViewGroup layout = (ViewGroup) inflater.inflate(customPagerEnum.getLayoutResId(), collection, false);
collection.addView(layout);
return layout;
}
@Override
public void destroyItem(ViewGroup collection, int position, Object view) {
collection.removeView((View) view);
}
@Override
public int getCount() {
return CustomPagerEnum.values().length;
}
@Override
public boolean isViewFromObject(View view, Object object) {
return view == object;
}
@Override
public CharSequence getPageTitle(int position) {
CustomPagerEnum customPagerEnum = CustomPagerEnum.values()[position];
return mContext.getString(customPagerEnum.getTitleResId());
}
}
There’s a lot going on here, but don’t fret. Step by step, here’s each piece of the puzzle:
isViewFromObject
method. Both refer to a particular item in the PagerAdapter. In this case, it’s a View.instantiateItem
method.While the PagerAdapter consists of many small pieces, each piece serves a unique and simple purpose.
So now, if you need a ViewPager full of static content but don’t want the heavy-handedness of Fragments, you have a nifty guide to using a ViewPager and PagerAdapter dedicated to displaying solely Views. Enjoy life without Fragments!
Introduction When computer programming was young, code was punched into cards. That is, holes were punched into a piece of cardboard in a format...
Jetpack Compose is a declarative framework for building native Android UI recommended by Google. To simplify and accelerate UI development, the framework turns the...
Big Nerd Ranch is chock-full of incredibly talented people. Today, we’re starting a series, Tell Our BNR Story, where folks within our industry share...