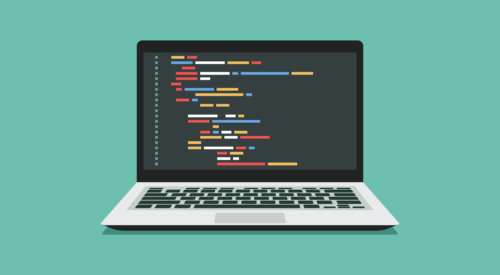
From Punched Cards to Prompts
AndroidIntroduction When computer programming was young, code was punched into cards. That is, holes were punched into a piece of cardboard in a format...
For as long as Android development has been around, we’ve been using setContentView
to inflate layouts in our Activity classes. And since the introduction of Fragments, we’ve had to override onCreateView
and use a layout inflater to get our view from a layout ID. They’re so common to write that it’s easy to not think about them much. At the same time, they feel a bit like boilerplate, right? Wouldn’t it be nice if there was a simpler way to inflate our views?
I’m glad to say that with a few recent AndroidX library updates, there is a simpler way.
During development of AndroidX Fragment 1.1.0 and AndroidX Activity 1.0.0, the kind folks at Google added a new way to inflate layouts. To start using these, add the following lines to the dependency block of your module-level build.gradle
file:
implementation 'androidx.appcompat:appcompat:1.1.0-rc01' // this is needed to use the updated AppCompatActivity implementation 'androidx.activity:activity-ktx:1.0.0-rc01' // or remove -ktx if not using kotlin implementation 'androidx.fragment:fragment-ktx:1.1.0-rc04' // or remove -ktx if not using kotlin
Note that these versions are the latest as of this writing, but update them as needed. You can find the latest versions here.
These new versions add the ability to pass the layout ID to the constructor of the Activity or Fragment base class, and it will handle inflation behind-the-scenes. So, instead of something like:
class MyActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.my_activity_layout) } // ... }
… you can instead write:
class MyActivity : AppCompatActivity(R.layout.my_activity_layout) { // ... }
This works similarly with Fragments as well. Make sure to import AndroidX Fragments (androidx.fragment.app.Fragment
) rather than the framework version (android.app.Fragment
).
Old way:
class MyFragment : Fragment() { override fun onCreateView(inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle?): View? { return layoutInflater.inflate(R.layout.my_fragment_layout, container, false) } // ... } ```
New way:
class MyFragment : Fragment(R.layout.my_fragment_layout) { // ... }
If the only code that was inside of the Activity’s onCreate
or the Fragment’s onCreateView
method was layout inflation code (like in the examples above), then you can completely remove those overridden methods. This is especially useful in Fragments, since you can put any logic for after the view is inflated in the onViewCreated
method.
This new way of using layouts isn’t going to change the landscape of UI development on Android (I’m lookin’ at you, Jetpack Compose), but it’s a small step that can save us from writing a little bit of extra code when making our Activities and Fragments, which I can certainly appreciate.
Introduction When computer programming was young, code was punched into cards. That is, holes were punched into a piece of cardboard in a format...
Jetpack Compose is a declarative framework for building native Android UI recommended by Google. To simplify and accelerate UI development, the framework turns the...
Big Nerd Ranch is chock-full of incredibly talented people. Today, we’re starting a series, Tell Our BNR Story, where folks within our industry share...